Introducing Adafruit Feather RP2040
Where I begin to work with the Adafruit Feather RP2040 (Feather) and MicroPython (uP) and use the Labrador to test the board.
Using Blink Test to Confirm Board Works
Using an Interactive Pin Test to Confirm Header Works
Sources
- Adafruit Feather RP2040
- Raspberry Pi RP2040 Getting Started
- RP2040 Datasheet
- Arm: Raspberry Pi RP2040: Our Microcontroller for the Masses
- Get Started with MicroPython on Raspberry Pi Pico
- Raspberry Pi Pico Python SDK
- MicroPython RP2 Docs
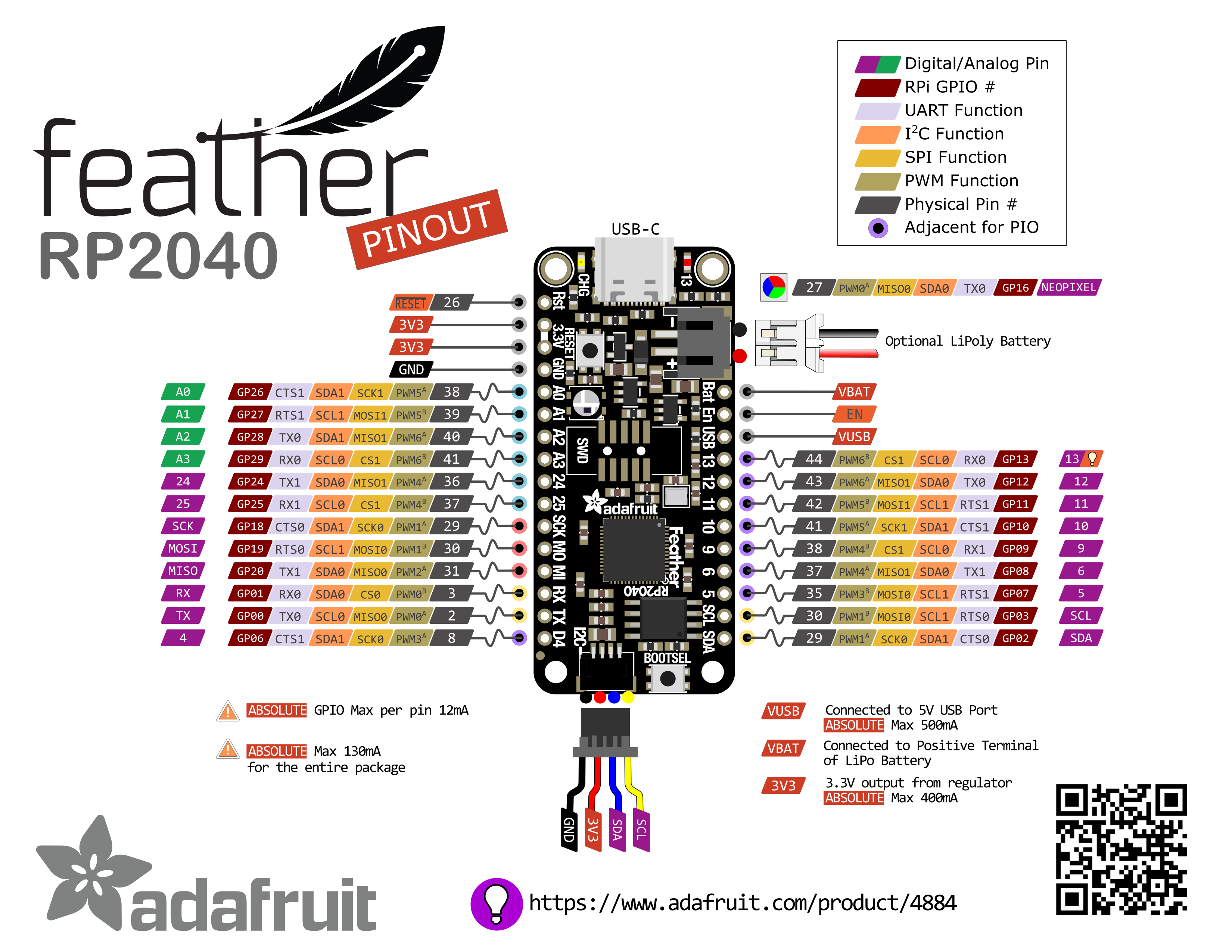
Background
After being disappointed with the uP development for the ESP32, I thought it would be fun to try the latest and greatest, hottest, just out of design, the Raspberry Pi RP2040 Microcontroller. I was able to secure two Adafruit Feather versions and will use those to test.
I am quite impressed with the level of documentation. This is something that the Raspberry Pi Foundation was quite strong about with the launch of the chip. The documentation would be available on the first day as well, and the documentation would be first-rate.
Getting Started
Clearly there has been a little more thought as to how to make it easy to install uP in the last few years. While the steps I followed for the ESP32 were absurdly easy, the equivalent for uP and the Feather board were even easier. In fact, confirming my cable was working took more time than the actual process:
- Download the uP software, this is for the Raspberry Pi Pico, I’m going to test it with the Feather
- Connect the Feather using a UBS C data/power cable.
- Hold the Boot/Sel button down (this is the button by the QWIC connector, NOT the USB connector)
- Press/Release the Reset button (this is the button by the USB connector)
- RPI-RP2 will show up as a drive, drag the software from step 1 to the drive
- Open a serial monitor and press/release Reset and you will be in business!
If the screen is blank (mine was) press Ctrl-D and that old, familar uP interface will show up.
ManPinTest
Let’s try our usual program ManPinTest and use the Labrador to view the outcome. What is just lovely about the Feather boards, is that many of the pins, i.e; Pin13 == LED are the same from board to board. In this case, I had changed the parameters such that I got this response (I shortened the print statement, the new version is at the end of this entry.):
Pin13 with 3.333333Hz freq & duty of 33.33334%
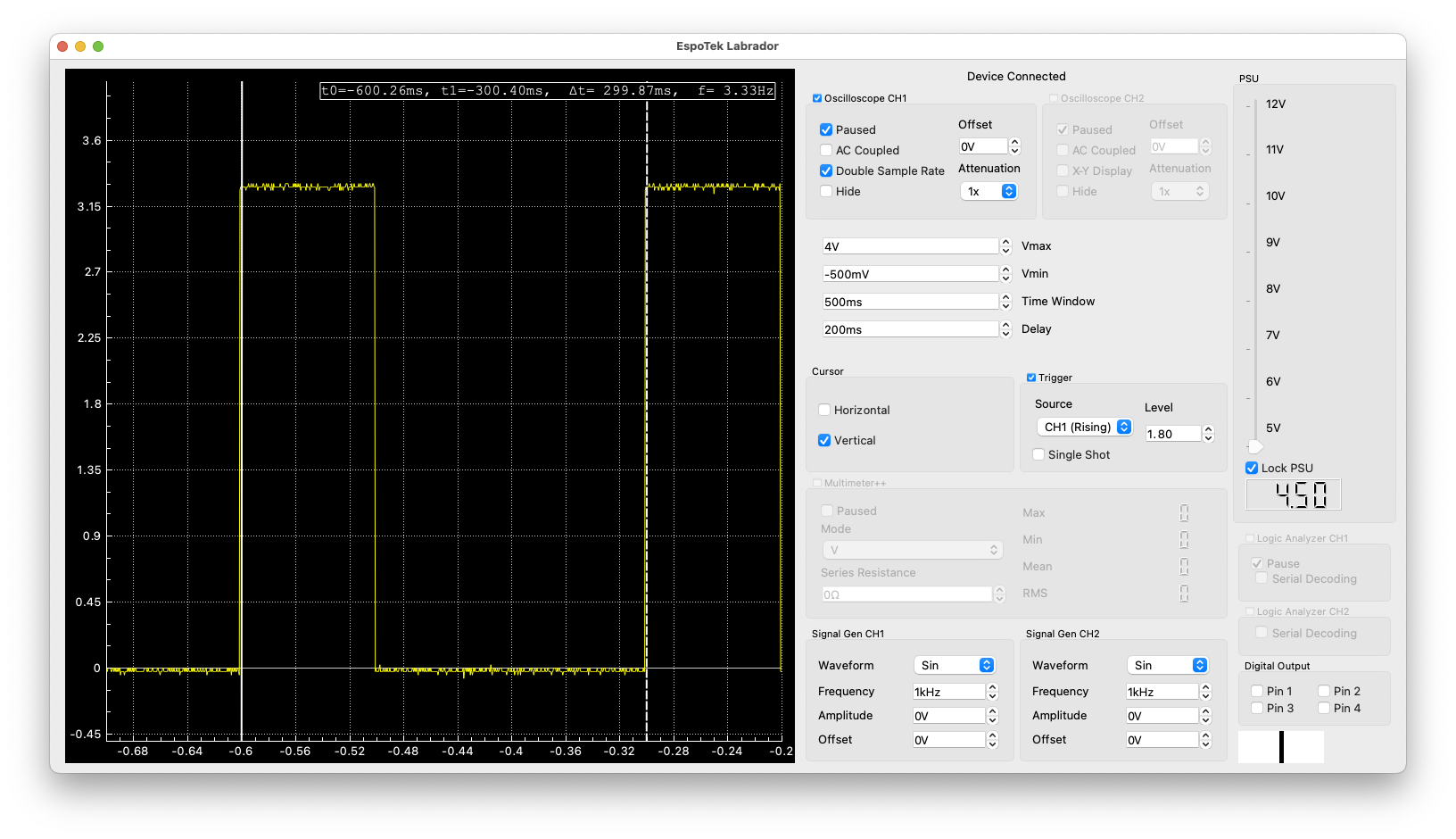
PinTest
Let’s try PinTest and see if it works. Works, in fact it worked so well, I went down the sides of the board and confirmed that each pin worked as expected. Well, everything worked until I got to pin0. I had a bug where I was checking for pins > 0 (line 69), and it needed to be >= 0. Fixed it and everything worked! So my soldering worked! :)
Here is a sample of the tests I ran. I would download PinTest using Ctrl-E/CMD-V/Ctrl-D then quickly move the Oscilloscope CH1 jumper from pin to pin. I’d press 1 and watch the CH1 on the Labrador scope, go HIGH, press 2, go LOW then see a pulse when I pressed 3. Once I had seen all three responses, I press 0 and go to the next pin.
Running Pin Test
Tests: 0=> new pin 1=> High 2=> Low 3=> Blink once
Enter pin to test: 28
28 enabled as Output
Enter test to run: 1
Run test 1
Pin(28, mode=OUT) is now High
Enter test to run: 2
Run test 2
Pin(28, mode=OUT) is now LOW
Enter test to run: 3
Run test 3
Pin(28, mode=OUT) will blink once
Enter test to run: 0
Run test 0
Running Pin Test
Tests: 0=> new pin 1=> High 2=> Low 3=> Blink once
Enter pin to test: 27
27 enabled as Output
Enter test to run: 1
Run test 1
Pin(27, mode=OUT) is now High
Enter test to run: 2
Run test 2
Pin(27, mode=OUT) is now LOW
Enter test to run: 3
Run test 3
Pin(27, mode=OUT) will blink once
Enter test to run: 0
Code
ManPinTestv2
# ManPinTest.py - blink a defined pin, print freq, duty cycle
# identify the pin to be tested
# either by pin i.e; 27
import machine
import time
use_pin = 13
pin = machine.Pin(use_pin, machine.Pin.OUT)
on_sleep = 100
off_sleep = 200
freq = 1 / (on_sleep + off_sleep) * 1000
duty = on_sleep / (on_sleep + off_sleep) * 100
print("Pin", use_pin, " with ", freq, "Hz freq & duty of ", duty, "%", sep='')
while 1 == 1:
pin.value(1)
time.sleep_ms(on_sleep)
pin.value(0)
time.sleep_ms(off_sleep)
PinTestv2 (fixed 0 pin bug, and other cleanup)
# PinTest.py - interactive app to test pins on a board
import machine
import time
minTest = 1
maxTest = 3
maxPins = 29
def getPin():
pin = int(input("Enter pin to test: "))
if (pin > maxPins):
print("Error, pin requested", pin,
" > number of output pins:", maxPins)
pin = -1
elif (pin < 0):
print("Error, pin requested", pin, " < 0:")
pin = -1
else:
print(pin, " enabled as Output")
return(pin)
def getTest():
test = int(input("Enter test to run: "))
if test > maxTest:
print("Error, test requested", test, "> tests allowed ")
test = -1
elif (test == 0):
print("Restarting")
elif test < minTest:
print("Error, test requested", test, "< 0")
test = -1
return(test)
def blink(pin):
pin.value(1) # sets the digital pinN on
time.sleep_ms(50) # waits for a second
pin.value(0) # sets the digital pinN on
time.sleep_ms(50) # waits for a second
def runTest_1(pin):
pin.value(1)
print(pin, " is High")
def runTest_2(pin):
pin.value(0)
print(pin, " is LOW")
def runTest_3(pin):
print(pin, " will blink once")
blink(pin)
def PinTest():
startState = True
testState = True
print("Running Pin Test")
print("""Tests: 0=> new pin 1=> High 2=> Low 3=> Blink once""")
while startState:
pin = getPin()
if pin >= 0:
startState = False
pinT = machine.Pin(pin, machine.Pin.OUT)
while testState:
test = getTest()
if test >= 0:
if test == 0:
startState = True
testState = False
elif test == 1: # 1 - set pin HIGH
runTest_1(pinT)
testState = True
elif test == 2: # 1 - set pin LOW
runTest_2(pinT)
testState = True
elif test == 3: # 1 - set pin to BLINK
runTest_3(pinT)
testState = True
else: # print error, not a valid test number
print(test, "entered. Must be 0, 1, 2, or 3")
testState = True
if __name__ == '__main__':
while 1 == 1:
PinTest()
Comments powered by Talkyard.