Developing in C for the ATmega328P: Function - digitalRead()
Where I describe the function - digitalRead().
Introduction
The function digitalRead() is analogous to digitalWrite(), in the sense, it takes a pin number as a parameter and as compared to setting its value, it returns the pin’s value. The value will either be a HIGH, indicating the value of the pin was approximately 5V or it will be LOW, indicating the value is approximately 0V or GND.
Background
Like digitalWrite(), it also requires using pinMode(), however there are two choices to use, INPUT or INPUT_PULLUP. For the most part, its best to use INPUT_PULLUP as it will have the least chance for error. What this means, is that when a pin is in INPUT_PULLUP, its value will always be HIGH unless it is specifically pulled low. For example, pressing a button connected to ground would pull the pin to GND or LOW. When using INPUT, you will need to ensure the device connected to the pin, will either be at 5V or GND.
Using digitalRead()
The image below illustrates a typical use of digitalRead(). A button is connected on one side to ground and the other side to a pin (pin 7). With pinMode(7, INPUT_PULLUP), digitalRead() will always return a HIGH until the button is pressed. Once the button is pressed, a connection between the pin 7 and GND is made, and digitalRead() will return a LOW. When LOW indicates an event has occured, it is called active-low and the logic to act must by designed accordingly. See code example.
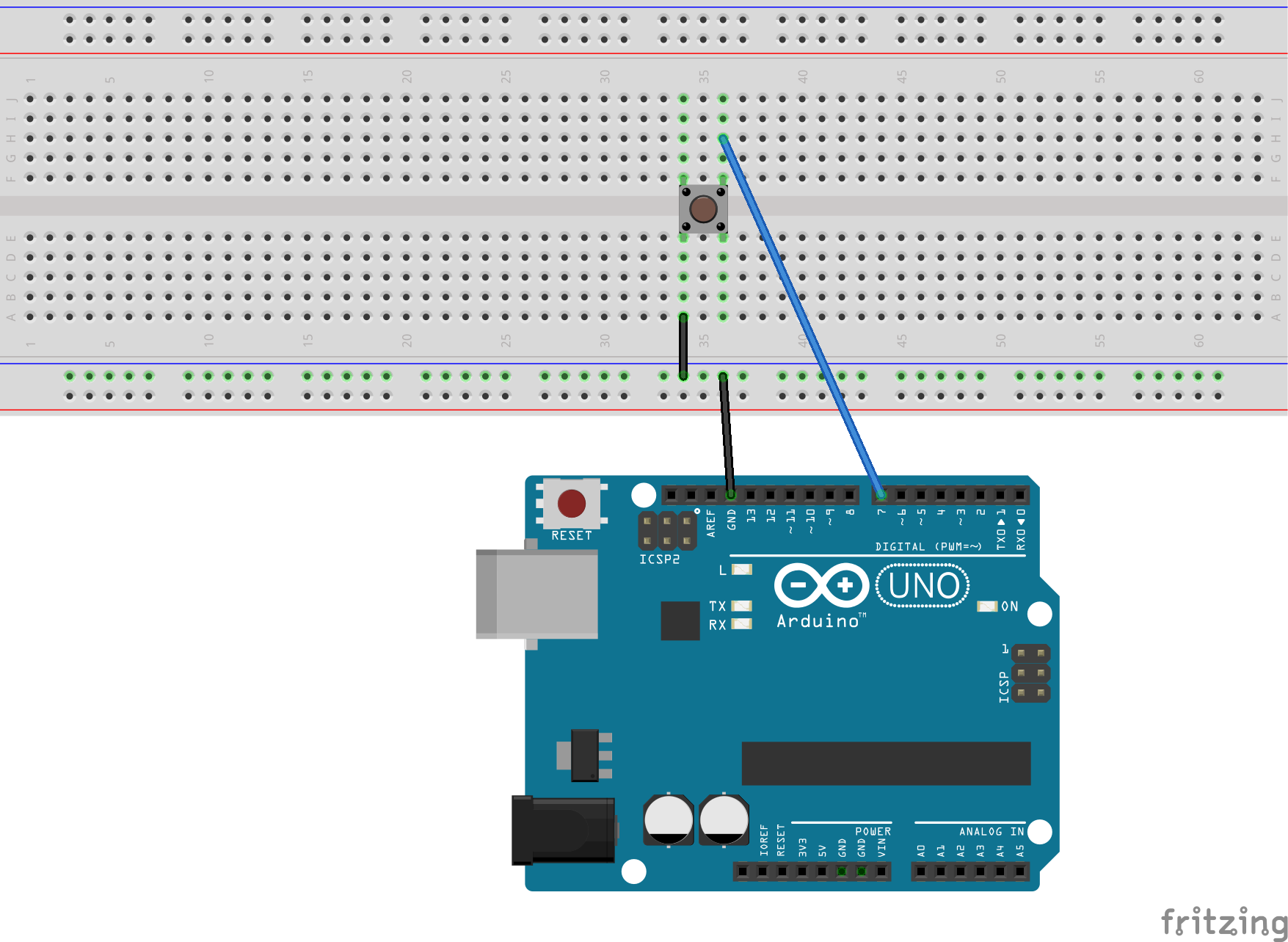
This code example will light the built-in LED, when the button is pressed. Once released, the LED will turn off. Line 7, is where we setup the pin and line 11 is where we check its value (active-low).
|
|
Comments powered by Talkyard.